这里介绍如何对NumPy数组进行索引、切片
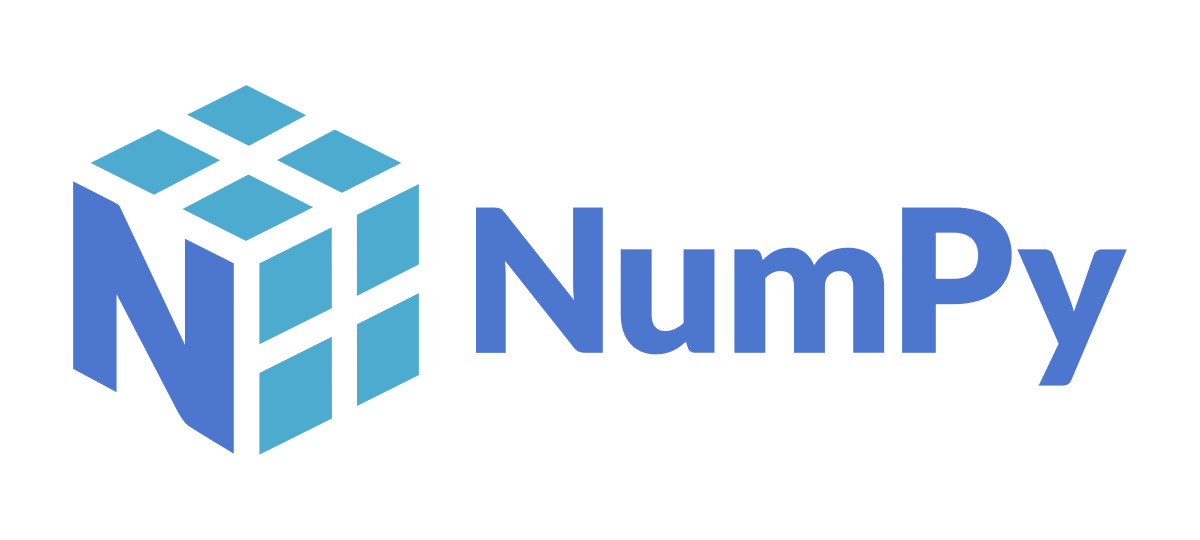
一维数组
对于一维数组而言,对其进行索引、切片、迭代。与Python列表的操作方式一样
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import numpy as np
a = np.array([2,4,6,8]) print(a)
print(f"a[0] -->> {a[0]}")
print(f"a[2] -->> {a[2]}")
print(f"a[0:2] --- {a[0:2]}")
print(f"a[-3:] --- {a[-3:]}")
for i in range( a.shape[0] ): print(f"i: {i} >>> {a[i]}")
|
多维数组
多维数组的索引、切片、迭代方式如下所示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| import numpy as np
a = np.arange(24).reshape(4,6) print(a)
print(f"a[0,3] -->> {a[0,3]}")
print(f"a[1,3] -->> {a[1,3]}")
print(f"a[2,-3] -->> {a[2,-3]}")
print(a[0:3, 1:4])
print(a[1:2, 2:3])
for i in a: print(f"i : {i}")
for j in a.flat: print(j, end=", ")
|
当某个轴的切片期望取该轴的全部数据,即完整切片。可使用 :冒号 来表示。特别地:当指定的切片少于轴的数量,则缺失的切片被视为完整切片
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| import numpy as np
a = np.arange(24).reshape(4,6) print(a)
print(a[1:3, :])
print(a[:,2:5])
print(a[3:5])
|
此外在指定切片时,还可以使用 …省略号 来表示缺失的 全部:冒号 。显然,省略号在指定切片时只能使用一次
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| import numpy as np
x = np.arange(16).reshape(2,2,2,2) print(x)
print(x[0,1,...])
print(x[...,0])
print(x[...,0,:,0])
|
索引数组
索引时,可以使用索引数组(索引值组成的数组)来获取多个元素
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| import numpy as np
square_nums = np.arange(9) ** 2 print(square_nums)
index1 = [3,1,7,2,1] print(square_nums[index1])
index2= np.array([3,1,7,2,1]) print(square_nums[index2])
|
当被索引数组为多维数组时,可通过 由多个索引数组组成的元组 来实现依次为各维度指定索引。此时要求各维度的索引数组形状必须相同
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| import numpy as np
a = np.arange(12).reshape(4,3) print(a)
rowIndex = np.array( [[0,2],[1,3]] ) print(rowIndex)
print(a[rowIndex])
colIndex = np.array([[0,0],[1,2]]) print(colIndex)
print(a[rowIndex, colIndex])
|
布尔索引
布尔数组形状 与 被索引数组形状 一致
NumPy当中支持使用具有相同形状的布尔数组作为索引。其中:True值表示选择相应位置处的元素;False值表示不选择相应位置处的元素。最终结果会将选择的元素展平为一维数组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import numpy as np
num = np.array( [ [13,-9,12], [-37,-92,22] ] ) print(num)
positive_index = num > 0 print(positive_index)
positive_num = num[positive_index] print(positive_num)
|
布尔索引用于赋值操作时,会将布尔数组中True值相应位置处的元素赋值为指定值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import numpy as np
num = np.array( [ [13,-9,12], [-37,-92,22] ] ) print(num)
negative_index = num < 0 print(negative_index)
num[negative_index] = 996 print(num)
|
一维布尔数组 索引 指定维度
一维的布尔数组 可以作为被索引数组的某个维度的索引,显然需要保证 该一维布尔数组的长度 与 被索引数组在指定维度上的长度 完全一样
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| import numpy as np
arr = np.array( [ [1, 2], [3, 4], [5, 6] ] )
b1 = np.array([True, False, True]) r1 = arr[b1,:]
r2 = arr[b1]
print(r1)
print(r2)
b3 = np.array([True, False]) r3 = arr[:, b3] print(r3)
|