这里介绍Python的模块
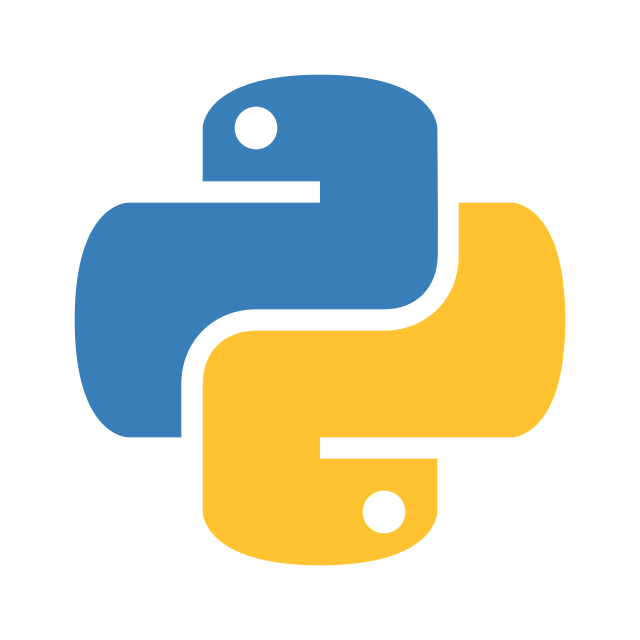
导入模块中函数
在Python中,一个py源文件即为一个模块。如下所示,有下述两个py文件

其中,hello.py文件的内容如下所示
1 2 3 4 5
| def hello_world(): print("Hello World")
def hello_person(name): print("Hello",name)
|
如果我们期望在main.py文件使用hello模块中的函数,可以通过下述手段实现
1 2 3 4 5 6
| import hello
hello.hello_world() hello.hello_person("Aaron")
|
1 2 3 4 5 6
| import hello as hi
hi.hello_world() hi.hello_person("Aaron")
|
1 2 3 4 5
| from hello import hello_world, hello_person
hello_world() hello_person("Aaron")
|
1 2 3 4
| from hello import hello_person as hip
hip("Aaron")
|
1 2 3 4 5 6
|
from hello import *
hello_world() hello_person("Aaron")
|
导入模块中类
现在我们来体现如何导入其他模块定义的类,实现为我所用。有下述两个py文件
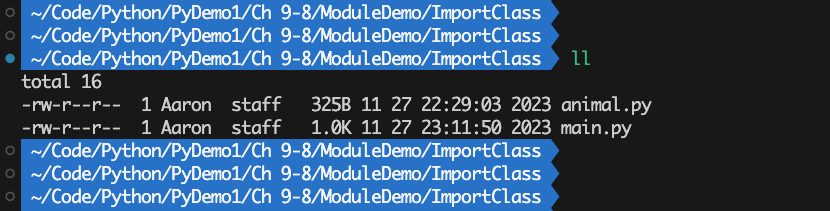
其中,animal.py文件的内容如下所示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class Cat: """ 猫 """ def __init__(self, name): self.name = name def say(self): print(self.name, "正在喵喵喵")
class Dog: """ 猫 """ def __init__(self, name): self.name = name def say(self): print(self.name, "正在狗叫: 汪汪汪")
|
如果我们期望在main.py文件使用animal模块中的类,可以通过下述手段实现
1 2 3 4 5 6 7 8
| import animal
my_cat1 = animal.Cat("Tom") my_cat1.say() my_dog1 = animal.Dog("Lucy") my_dog1.say()
|
1 2 3 4 5 6 7
| import animal as ani
my_cat2 = ani.Cat("Tom") my_cat2.say() my_dog2 = ani.Dog("Lucy") my_dog2.say()
|
1 2 3 4 5 6 7
| from animal import Cat, Dog
my_cat3 = Cat("Tom") my_cat3.say() my_dog3 = Dog("Lucy") my_dog3.say()
|
1 2 3 4 5
| from animal import Dog as doooog
my_dog4 = doooog("Lucy") my_dog4.say()
|
1 2 3 4 5 6 7 8
|
from animal import *
my_cat5 = Cat("Tom") my_cat5.say() my_dog5 = Dog("Lucy") my_dog5.say()
|
参考文献
- Python编程·第3版:从入门到实践 Eric Matthes著
- Python基础教程·第3版 Magnus Lie Hetland著
- 流畅的Python·第1版 Luciano Ramalho著