这里就Groovy中常用注解进行说明
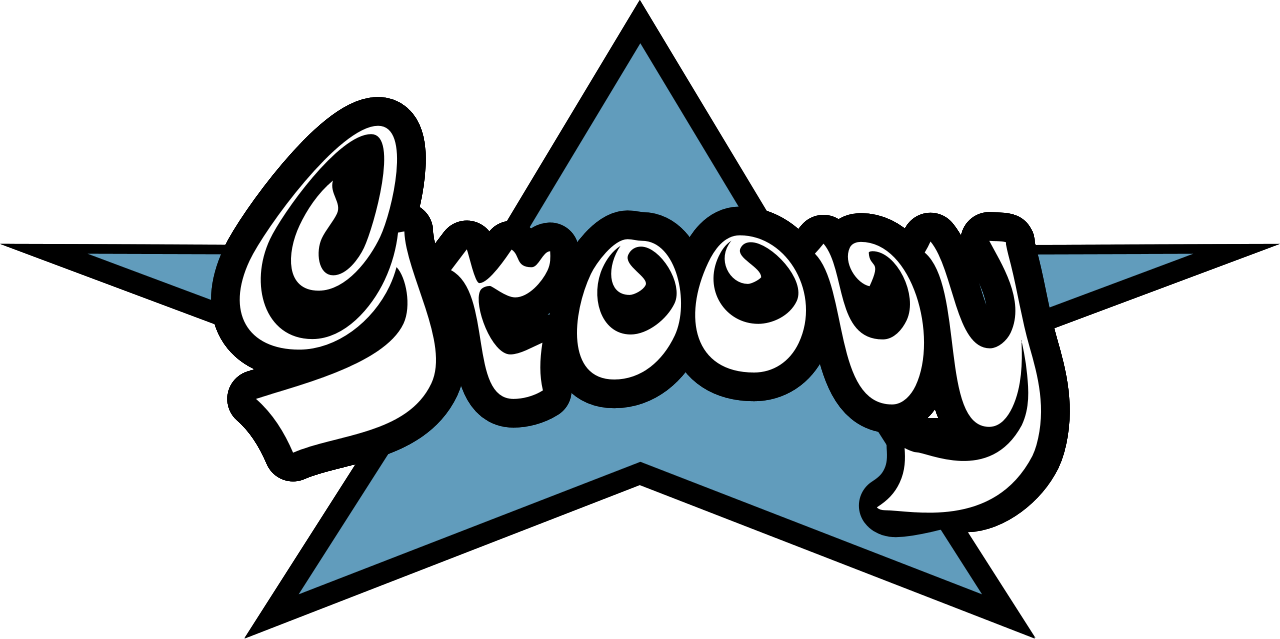
类的常用方法
在OOP中,类的常用方法包括toString、equals、hashcode方法、构造器。为此Groovy中提供了@Canonical注解,该注解实际上是组合了@ToString、@EqualsAndHashCode、@TupleConstructor 注解。用于生成类的toString、equals、hashcode方法、构造器。故我们也可以不使用@Canonical注解,而是按需选用下列注解
- @ToString:生成toString方法
- @EqualsAndHashCode:生成equals, hashcode方法
- @TupleConstructor:生成构造器
而@Builder注解则可以很方便地帮我们生成支持链式调用的构造器,示例代码如下所示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| class GenerateDemo { static void testConstructor() { Pc pc1 = new Pc()
Pc pc2 = new Pc("Dell") Pc pc3 = new Pc("Dell", 3) Pc pc4 = new Pc("Dell", 3, 1999d)
Pc pc5 = new Pc(brand: "HP") Pc pc6 = new Pc(num:5, price: 288.99d)
Pc pc7 = Pc.builder() .price(5999) .brand("ThinkPad") .num(34) .build(); }
static void testOther() { Pc pc1 = new Pc("Dell",2,200) Pc pc2 = new Pc("Dell",2,200)
assert pc1 !== pc2 assert pc1.hashCode() == pc2.hashCode() assert pc1 == pc2 assert pc1.equals(pc2)
assert pc1.toString() == "com.aaron.AnnotationDemo.Pc(Dell, 2, 200.0)" } }
@Canonical @Builder class Pc { String brand
Integer num;
Double price; }
|
单例模式
通过@Singleton注解则可以快速实现单例模式,示例代码如下所示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class GenerateDemo { static void testSingleton() { RedisClient redisClient1 = RedisClient.instance RedisClient redisClient2 = RedisClient.instance
assert redisClient1.ip == "127.0.0.1" assert redisClient1.port == 6379
assert redisClient1 === redisClient2 } }
@Singleton(lazy = true) class RedisClient { String ip = "127.0.0.1"
Integer port = 6379 }
|
参考文献
- Groovy In Action · 2nd Edition Dierk König、Guillaume Laforge著