MyBatis,作为目前流行的ORM框架,大大方便了日常开发。而对于分页查询,虽然可以通过SQL的limit语句实现,但是比较繁琐。而MyBatis PageHelper的出现,则解决了这一痛点。这里将介绍如何在Spring Boot、MyBatis的环境中通过MyBatis PageHelper高效方便的实现分页查询
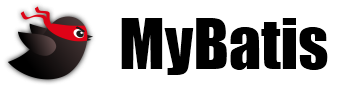
配置
1. 添加Maven依赖
1 2 3 4 5 6
| <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper-spring-boot-starter</artifactId> <version>1.2.5</version> </dependency>
|
2. 添加配置
在application.properties配置文件中添加MyBatis PageHelper的配置项
1 2 3 4 5
| pagehelper.helperDialect=mysql pagehelper.reasonable=true pagehelper.supportMethodsArguments=true pagehelper.params=count=countSql
|
分页查询
通过 MyBatis PageHelper 进行分页查询实际上非常简单,只需在service(或mapper)方法执行查询前,调用一次 PageHelper.startPage(pageNum,pageSize) 来设置分页查询参数即可,其中pageNum 为记录页数,pageSize 为单页记录数量。此时service(或mapper)方法的查询结果就是分页后的结果了。如果期望获得相关的分页信息,还可以将查询结果封装到PageInfo对象中,以获得总页数、总记录数、当前页数等相关分页信息
现在通过一个实际示例,来具体演示操作,这里我们提供了一个分页查询的Controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
|
@ResponseBody @RequestMapping("/findPage") public List<Student> findPage(@RequestParam int pageNum, @RequestParam int pageSize) { PageHelper.startPage(pageNum,pageSize); List<Student> studentList = studentService.findList();
for(Student student : studentList) { System.out.println("element : " + student); }
PageInfo pageInfo = new PageInfo( studentList ); System.out.println("总页数: " + pageInfo.getPages()); System.out.println("总记录数: " + pageInfo.getTotal()); System.out.println("当前页数: " + pageInfo.getPageNum()); System.out.println("当前页面记录数量: " + pageInfo.getSize());
return pageInfo.getList(); }
|
service方法中所调用的查询SQL如下所示,可以看到,SQL中无需使用limit语句
1 2 3 4 5 6 7 8 9 10 11 12
| ... <resultMap id="studentResultMap" type="com.aaron.springbootdemo.pojo.Student"> <id property="id" column="id" jdbcType="INTEGER"/> <result property="username" column="username" jdbcType="VARCHAR"/> <result property="sex" column="sex" jdbcType="VARCHAR"/> <result property="address" column="address" jdbcType="VARCHAR"/> </resultMap>
<select id="findList" parameterType="String" resultMap="studentResultMap"> SELECT * FROM user </select> ...
|
测试结果如下所示
查询第一页:
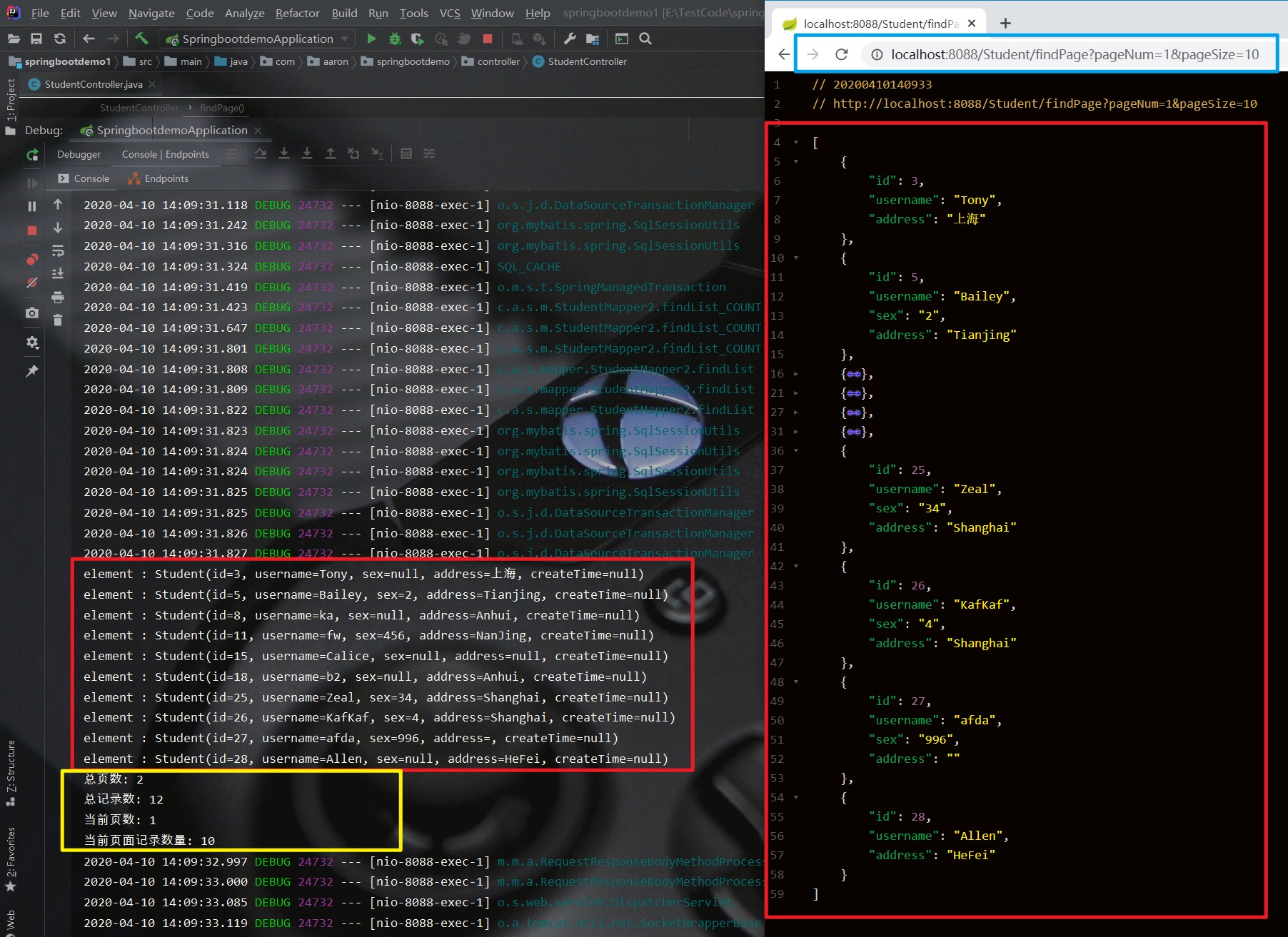
查询第二页:
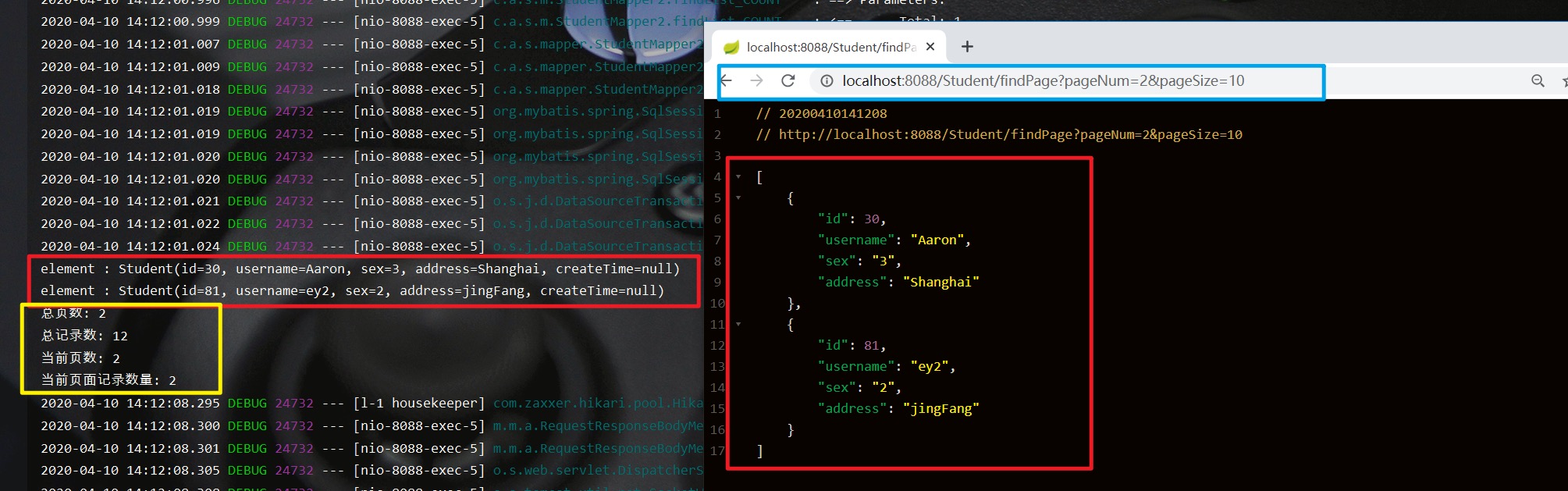
Note:
PageHelper.startPage(pageNum,pageSize) 只对其后的第一次SQL查询进行分页。故若需进行分页查询,必须每次在service(或mapper)方法执行SQL查询前调用PageHelper.startPage(pageNum,pageSize) 方法