这里介绍如何在运行时修改注解的值
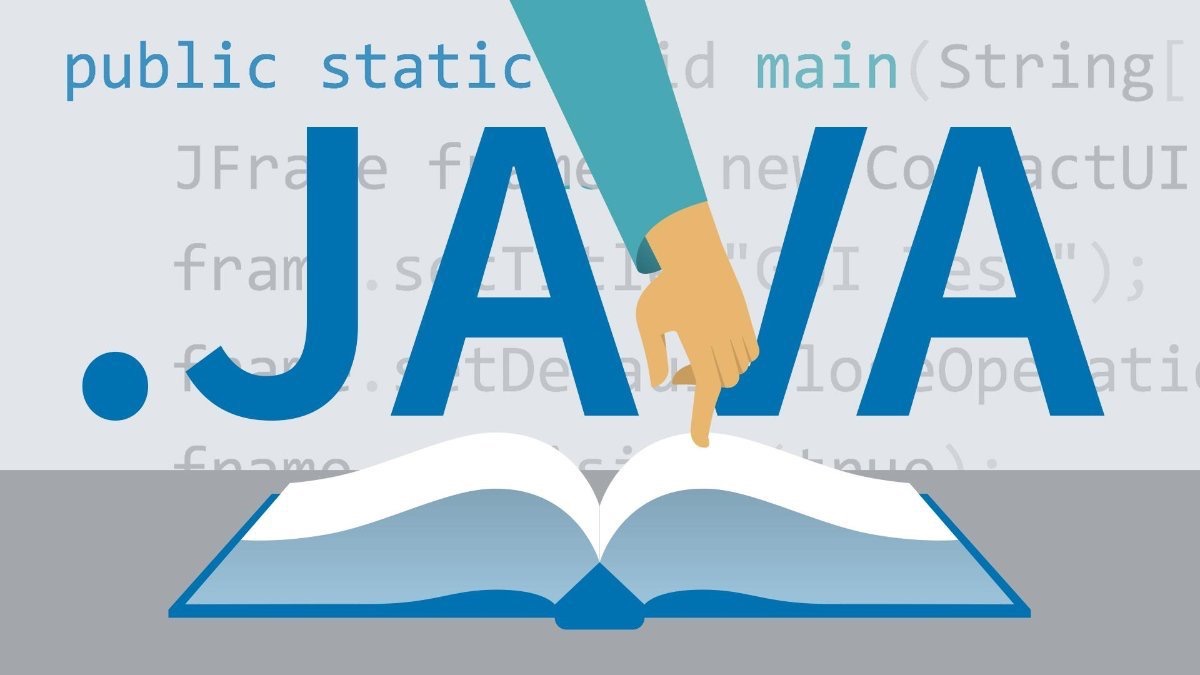
基本原理
查看JDK中Annotation接口的注释,说明所有注解都扩展自Annotation接口。换言之,注解本质上就是一个继承了Annotation的接口
1 2 3 4 5 6 7 8 9 10 11
| package java.lang.annotation;
public interface Annotation { ... }
|
这里,我们先自定义一个注解@MyLog,同时定义一个类UserInfoService来使用该注解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
|
@Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface MyLog { String level() default "TRACE"; }
...
public class UserInfoService {
@MyLog( level = "DEBUG") public void attachById(Integer userId) { }
@MyLog( level = "INFO") public void batchDelete(List<Integer> ids) {
} }
|
现在我们通过debug的方式来查看反射后的注解对象。由于注解的具体实现类是利用JDK动态代理生成的。故我们通过反射获得的注解对象,实际上是运行时生成的动态代理对象Proxy
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class RuntimeModifyAnnoTest { public static void main(String[] args) throws NoSuchMethodException { test1(); }
public static void test1() throws NoSuchMethodException { Method attachByIdMethod = UserInfoService.class.getDeclaredMethod("attachById", Integer.class); MyLog attachByIdMyLog = attachByIdMethod.getDeclaredAnnotation( MyLog.class ); String level = attachByIdMyLog.level(); System.out.println("Level : " + level); } }
|
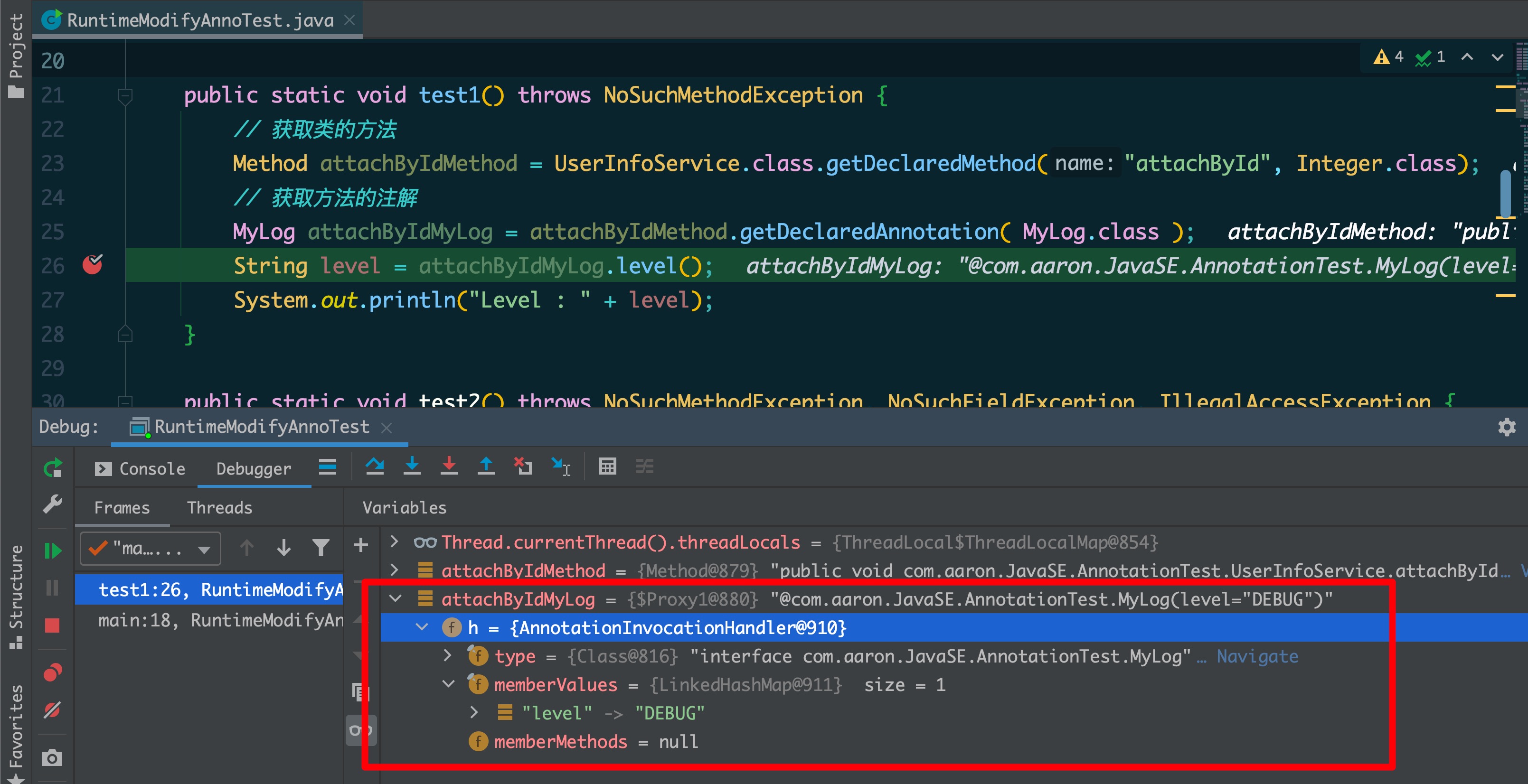
而当我们通过这个动态代理对象Proxy访问level属性值时,其会通过调用 AnnotationInvocationHandler 的invoke方法来实现。进一步地观察invoke方法的源码,不难看出其最终是从memberValues这个Map中获取到对应的值
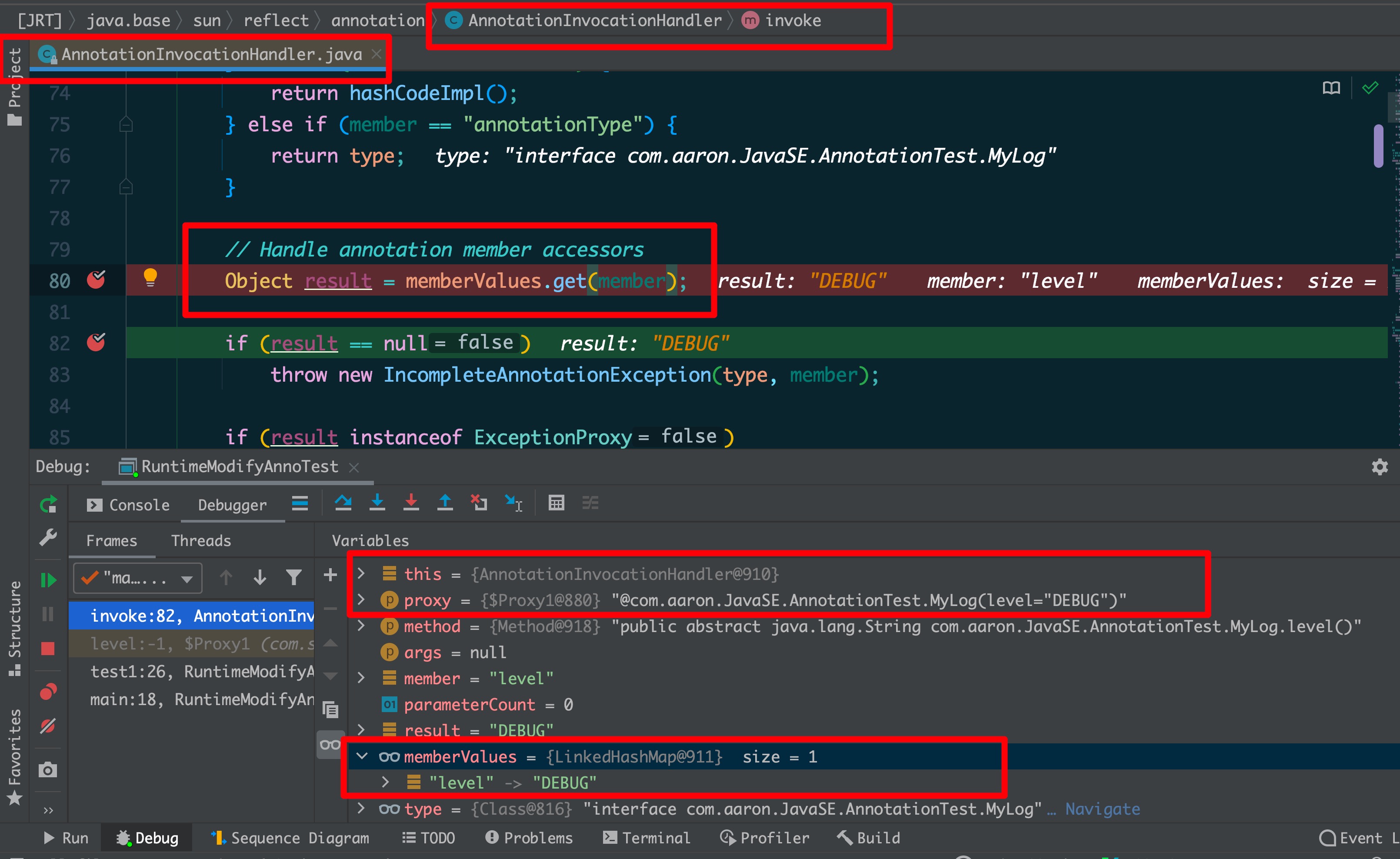
实践
现在,我们如果期望在运行时修改注解的属性值就非常简单了。只需先通过反射获取注解的代理对象,然后获取该代理对象的InvocationHandler实例,最后修改AnnotationInvocationHandler实例的memberValues字段中属性值即可。下述代码,尝试将attachById方法上@MyLog注解的值由DEBUG修改为WARN;而batchDelete方法上@MyLog注解的值则不会收到影响
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
|
public class RuntimeModifyAnnoTest {
public static void main(String[] args) throws NoSuchMethodException, NoSuchFieldException, IllegalAccessException { test2(); }
public static void test2() throws NoSuchMethodException, NoSuchFieldException, IllegalAccessException { Method attachByIdMethod = UserInfoService.class.getDeclaredMethod("attachById", Integer.class); MyLog attachByIdMyLog = attachByIdMethod.getDeclaredAnnotation( MyLog.class ); String level = attachByIdMyLog.level(); System.out.println("attachById方法 @MyLog注解 level属性值: " + level);
InvocationHandler invocationHandler = Proxy.getInvocationHandler( attachByIdMyLog ); Field memberValuesField = invocationHandler.getClass().getDeclaredField("memberValues"); memberValuesField.setAccessible(true); Map<String, Object> memberValues = (Map<String, Object>) memberValuesField.get(invocationHandler);
memberValues.put("level", "WARN"); level = attachByIdMyLog.level(); System.out.println("attachById方法 @MyLog注解 level属性值: " + level);
Method batchDeleteMethod = UserInfoService.class.getDeclaredMethod("batchDelete", List.class); MyLog batchDeleteMyLog = batchDeleteMethod.getDeclaredAnnotation( MyLog.class ); level = batchDeleteMyLog.level(); System.out.println("batchDelete方法 @MyLog注解 level属性值: " + level); } }
|
效果如下所示
